L'exécutable
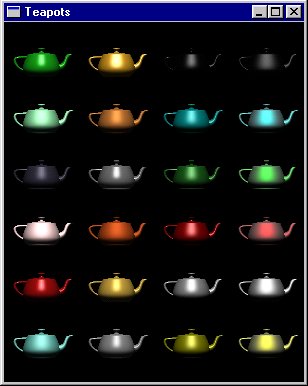
Le source: Teapots.cpp
#include "windows.h"
#include <GL/gl.h>
#include <GL/glu.h>
#include <GL/glaux.h>
void myinit(void) {
GLfloat ambient[] = { 0.0F,0.0F,0.0F,1.0F };
GLfloat diffuse[] = { 1.0F,1.0F,1.0F,1.0F };
GLfloat specular[] = { 1.0F,1.0F,1.0F,1.0F };
GLfloat position[] = { 0.0F,3.0F,3.0F,0.0F };
GLfloat lmodel_ambient[] = { 0.2F,0.2F,0.2F,1.0F };
GLfloat local_view[] = { 0.0F };
glLightfv(GL_LIGHT0,GL_AMBIENT,ambient);
glLightfv(GL_LIGHT0,GL_DIFFUSE,diffuse);
glLightfv(GL_LIGHT0,GL_POSITION,position);
glLightModelfv(GL_LIGHT_MODEL_AMBIENT,lmodel_ambient);
glLightModelfv(GL_LIGHT_MODEL_LOCAL_VIEWER,local_view);
glFrontFace (GL_CW);
glEnable(GL_LIGHTING);
glEnable(GL_LIGHT0);
glEnable(GL_AUTO_NORMAL);
glEnable(GL_NORMALIZE);
glEnable(GL_DEPTH_TEST);
glDepthFunc(GL_LESS);
}
void renderTeapot (GLfloat x,GLfloat y,GLfloat ambr,GLfloat ambg,GLfloat ambb,GLfloat difr,GLfloat difg,GLfloat difb,GLfloat specr,GLfloat specg,GLfloat specb,GLfloat shine) {
float mat[4];
glPushMatrix();
glTranslatef (x,y,0.0);
mat[0] = ambr;
mat[1] = ambg;
mat[2] = ambb;
mat[3] = 1.0;
glMaterialfv(GL_FRONT,GL_AMBIENT,mat);
mat[0] = difr;
mat[1] = difg;
mat[2] = difb;
glMaterialfv(GL_FRONT,GL_DIFFUSE,mat);
mat[0] = specr;
mat[1] = specg;
mat[2] = specb;
glMaterialfv(GL_FRONT,GL_SPECULAR,mat);
glMaterialf(GL_FRONT,GL_SHININESS,shine*128.0F);
auxSolidTeapot(1.0);
glPopMatrix();
}
void CALLBACK display(void) {
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
renderTeapot(2.0F,17.0F,0.0215F,0.1745F,0.0215F,0.07568F,0.61424F,0.07568F,0.633F,0.727811F,0.633F,0.6F);
renderTeapot(2.0F,14.0F,0.135F,0.2225F,0.1575F,0.54F,0.89F,0.63F,0.316228F,0.316228F,0.316228F,0.1F);
renderTeapot(2.0F,11.0F,0.05375F,0.05F,0.06625F,0.18275F,0.17F,0.22525F,0.332741F,0.328634F,0.346435F,0.3F);
renderTeapot(2.0F,8.0F,0.25F,0.20725F,0.20725F,1.0F,0.829F,0.829F,0.296648F,0.296648F,0.296648F,0.088F);
renderTeapot(2.0F,5.0F,0.1745F,0.01175F,0.01175F,0.61424F,0.04136F,0.04136F,0.727811F,0.626959F,0.626959F,0.6F);
renderTeapot(2.0F,2.0F,0.1F,0.18725F,0.1745F,0.396F,0.74151F,0.69102F,0.297254F,0.30829F,0.306678F,0.1F);
renderTeapot(6.0F,17.0F,0.329412F,0.223529F,0.027451F,0.780392F,0.568627F,0.113725F,0.992157F,0.941176F,0.807843F,0.21794872F);
renderTeapot(6.0F,14.0F,0.2125F,0.1275F,0.054F,0.714F,0.4284F,0.18144F,0.393548F,0.271906F,0.166721F,0.2F);
renderTeapot(6.0F,11.0F,0.25F,0.25F,0.25F,0.4F,0.4F,0.4F,0.774597F,0.774597F,0.774597F,0.6F);
renderTeapot(6.0F,8.0F,0.19125F,0.0735F,0.0225F,0.7038F,0.27048F,0.0828F,0.256777F,0.137622F,0.086014F,0.1F);
renderTeapot(6.0F,5.0F,0.24725F,0.1995F,0.0745F,0.75164F,0.60648F,0.22648F,0.628281F,0.555802F,0.366065F,0.4F);
renderTeapot(6.0F,2.0F,0.19225F,0.19225F,0.19225F,0.50754F,0.50754F,0.50754F,0.508273F,0.508273F,0.508273F,0.4F);
renderTeapot(10.0F,17.0F,0.0F,0.0F,0.0F,0.01F,0.01F,0.01F,0.50F,0.50F,0.50F,.25F);
renderTeapot(10.0F,14.0F,0.0F,0.1F,0.06F,0.0F,0.50980392F,0.50980392F,0.50196078F,0.50196078F,0.50196078F,.25F);
renderTeapot(10.0F,11.0F,0.0F,0.0F,0.0F,0.1F,0.35F,0.1F,0.45F,0.55F,0.45F,.25F);
renderTeapot(10.0F,8.0F,0.0F,0.0F,0.0F,0.5F,0.0F,0.0F,0.7F,0.6F,0.6F,.25F);
renderTeapot(10.0F,5.0F,0.0F,0.0F,0.0F,0.55F,0.55F,0.55F,0.70F,0.70F,0.70F,.25F);
renderTeapot(10.0F,2.0F,0.0F,0.0F,0.0F,0.5F,0.5F,0.0F,0.60F,0.60F,0.50F,.25F);
renderTeapot(14.0F,17.0F,0.02F,0.02F,0.02F,0.01F,0.01F,0.01F,0.4F,0.4F,0.4F,.078125F);
renderTeapot(14.0F,14.0F,0.0F,0.05F,0.05F,0.4F,0.5F,0.5F,0.04F,0.7F,0.7F,.078125F);
renderTeapot(14.0F,11.0F,0.0F,0.05F,0.0F,0.4F,0.5F,0.4F,0.04F,0.7F,0.04F,.078125F);
renderTeapot(14.0F,8.0F,0.05F,0.0F,0.0F,0.5F,0.4F,0.4F,0.7F,0.04F,0.04F,.078125F);
renderTeapot(14.0F,5.0F,0.05F,0.05F,0.05F,0.5F,0.5F,0.5F,0.7F,0.7F,0.7F,.078125F);
renderTeapot(14.0F,2.0F,0.05F,0.05F,0.0F,0.5F,0.5F,0.4F,0.7F,0.7F,0.04F,.078125F);
glFlush();
}
void CALLBACK myReshape(int w,int h) {
glViewport(0,0,w,h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
if (w <= h)
glOrtho(0.0,16.0,0.0,16.0*(GLfloat)h/(GLfloat)w,-10.0,10.0);
else
glOrtho(0.0,16.0*(GLfloat)w/(GLfloat)h,0.0,16.0,-10.0,10.0);
glMatrixMode(GL_MODELVIEW);
}
void main(void) {
auxInitDisplayMode(AUX_SINGLE|AUX_RGB|AUX_DEPTH);
auxInitPosition(0,0,300,360);
auxInitWindow("Teapots");
myinit();
auxReshapeFunc(myReshape);
auxMainLoop(display);
}
RETOUR